Sum with forEach
#
const arr = [42, 666, 17];
let sum = 0;
arr.forEach(num => sum += num);
console.log(sum);
Sum with reduce
#
const arr = [42, 666, 17];
const sum = arr.reduce((total, num) => total + num, 0);
console.log(sum);
Sum of Points#
const arr = [
{ name: 'nisim', points: 42 },
{ name: 'shlomo', points: 666 },
{ name: 'david', points: 23 }
];
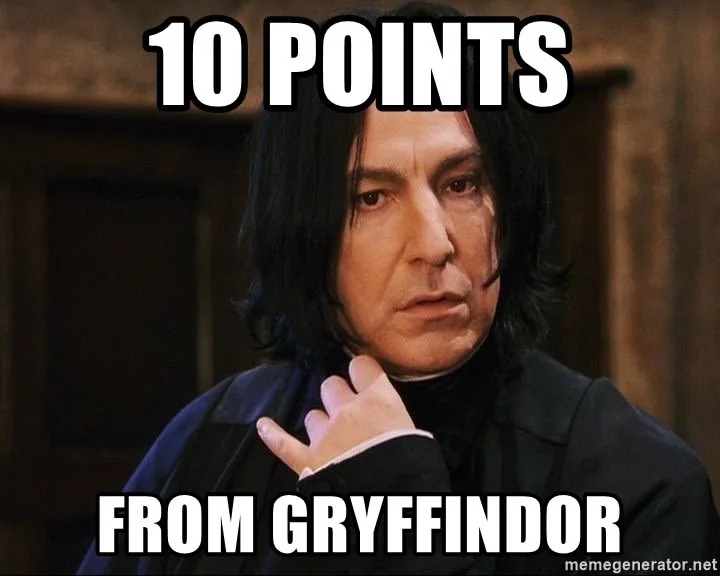
With forEach
#
let sum = 0;
arr.forEach(obj => sum += obj.points);
console.log(sum);
With reduce
#
const sum = arr.reduce((total, curObj) => total + curObj.points, 0);
console.log(sum);
Multiplication with forEach
#
const arr = [42, 666, 17];
let result = 1;
arr.forEach(num => result *= num);
console.log(product);
Multiplication with reduce
#
const arr = [42, 666, 17];
const result = arr.reduce((total, num) => total * num, 1);
console.log(result);
Sum of squares with forEach
#
const arr = [42, 666, 17];
let sum = 0;
arr.forEach(num => sum += (num ** 2));
console.log(sum);
Sum with reduce
#
const arr = [42, 666, 17];
const sum = arr.reduce((total, num) => total + (num ** 2) , 0);
console.log(sum);
Count Zeros with forEach
#
const arr = [1, 1, 0, 0, 0, 1, 0];
let count = 0;
arr.forEach(num => {
if (num === 0) count++;
});
console.log(count);
Count Zeros with reduce
#
const arr = [1, 1, 0, 0, 0, 1, 0];
const count = arr.reduce((total, num) => total + num === 0 ? 1 : 0, 0);
console.log(count);